Java: Removing Duplicate Elements from Array
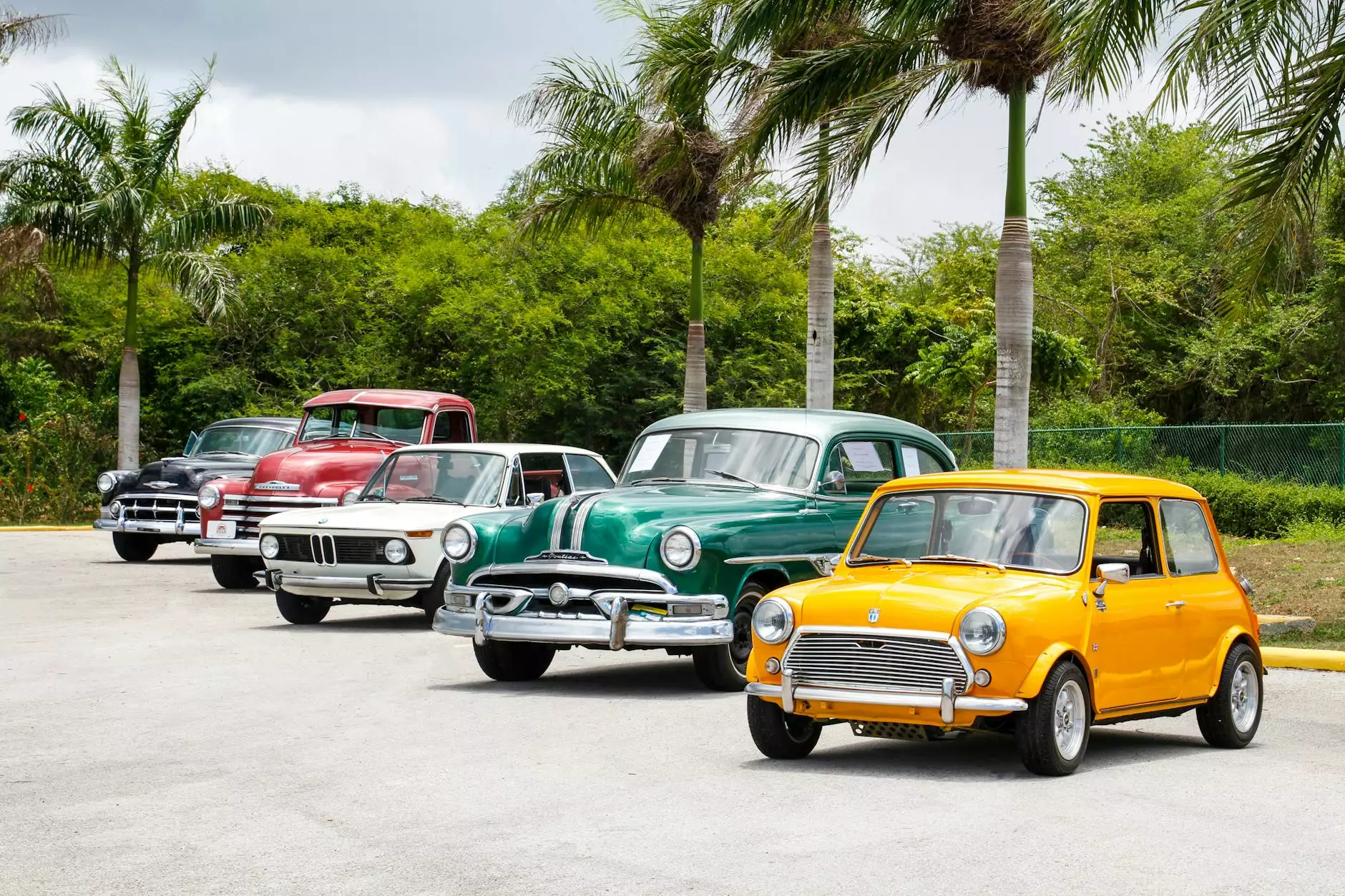
At LSTailors.com, we are dedicated to providing you with valuable insights and expert guidance on various aspects of Java programming. In today's article, we will delve into the topic of removing duplicate elements from an array in Java. This is a common task in many programming scenarios and understanding the most efficient approach can significantly improve your code's performance. Let's dive in!
The Importance of Removing Duplicate Elements
Removing duplicate elements from an array is a crucial step in data processing and analysis. It ensures that each element in the array is unique, allowing for accurate calculations, efficient searching, and better overall performance of your application.
Understanding the Problem
Before we jump into the implementation, let's establish a clear understanding of the problem. Consider the following scenario: you have an array containing various elements, and you want to remove any duplicates, keeping only the distinct values. How can we achieve this in Java?
Approach 1: Using a Set
One of the most straightforward approaches to remove duplicate elements from an array is by utilizing a Set. A Set is a collection that does not allow duplicate elements, making it a perfect candidate for our task.
Here's a code example:
import java.util.HashSet; import java.util.Set; public class ArrayUtils { public static int[] removeDuplicates(int[] array) { Set set = new HashSet(); for (int element : array) { set.add(element); } int[] uniqueArray = new int[set.size()]; int index = 0; for (int element : set) { uniqueArray[index++] = element; } return uniqueArray; } }In this implementation, we first create a Set called "set" to store the unique elements. Then, we iterate through the input array and add each element to the set using the add() method. Since Sets do not allow duplicates, only the distinct values will be added.
Next, we create a new array, uniqueArray, with the same size as the set. We iterate through the set and assign each element to the corresponding index in the unique array. Finally, we return the unique array as the result.
Approach 2: Using Brute Force
Another approach to remove duplicate elements from an array is by utilizing the brute force method. Although this method may not be as efficient as using a Set, it can still achieve the desired outcome.
Here's a code example:
public class ArrayUtils { public static int[] removeDuplicates(int[] array) { int n = array.length; int[] uniqueArray = new int[n]; int currentIndex = 0; for (int i = 0; iremoving duplicate elements from array in java 0789225888